SimpleZoom v.1.0.1
Overview
The SimpleZoom jQuery plugin creates a user controlled viewfinder element which is used to designate a region of the target image to be enlarged and then displayed within the plugins designated viewport element. By default the viewport and viewfinder are the same element creating the impression of a magnifying lens. Alternatively, a separate element may be specified as the active viewport.
SimpleZoom offers customization through various options, and automation through a simple API exposed as plugin methods and events.
SimpleZoom is open source software published under the MIT License.
Usage
Initializing the plugin
Consider an image element with an id
of "selector". The plugin is initialized as follows.
$("#selector").simpleZoom();
Initializing the plugin with options
The plugin may be invoked with an options
object containing properties to override the defaults.
The defaults may be accessed at $.fn.simpleZoom.defaults
.
$("#selector").simpleZoom({
zoom: 0.25 //set a 25% magnification
});
Compatibility
- Compatible with jQuery 2.1.0 in Mozilla Firefox, Google Chrome and Internet Explorer 9+.
- Compatible with jQuery 1.11.0 in Mozilla Firefox, Google Chrome and Internet Explorer 7+.
Installation
Include the following references in your web project. Note that the shown paths are relative to the package and should be edited as necessary.
<script type="text/javascript" src="js/jquery.simplezoom.js"></script>
<link href="css/simplezoom.css" type="text/css" rel="stylesheet" />
The package also contains img/transparent.png
referenced by the simplezoom-overlay
CSS class defined in css/simplezoom.css
. This resource is required for compatibility with IE7 and IE8.
Notes
- If the plugin target is a collection of elements then the plugin is only applied to the first element.
- In order to function properly the plugin requires additional CSS included in the download package.
Changelog
Version | Comment |
---|---|
1.0.1 | Construction is now correctly deferred until after the plugin returns. |
1.0.0 | Initial release. |
Plugin Options
Custom options may be specified at initialization or set using the option plugin method. Options which
are labeled as dynamic
can be set after initialization where as those labeled constant
cannot.
afterHide variable
Description
A callback to be invoked after the viewfinder is hidden. Note that all options specified callbacks will be invoked with this
set to be the plugin's target. See the afterHide event for more details.
Examples
Set option
Set the afterHide
option.
$("#selector").simpleZoom("option", "afterHide", function(){
this.show(); // this is the SimpleZoom instance
});
Unset option
To remove a callback set afterHide
to be a non-Function
.
$("#selector").simpleZoom("option", "afterHide", null);
afterShow variable
Description
A callback to be invoked after the viewfinder is shown. See the afterShow event for more details.
beforeDestroy variable
Description
A callback to be invoked before the plugin is destroyed. See the beforeDestroy event for more details.
beforeHide variable
Description
A callback to be invoked when the viewfinder hide animation begins. See the beforeHide event for more details.
beforeShow variable
Description
A callback to be invoked when the viewfinder show animation begins. See the beforeShow event for more details.
className.overlay constant
Description
The CSS class to be assigned to the element which overlays the plugin's target.
className.viewfinder constant
Description
The CSS class to be assigned to the viewfinder element.
className.viewport constant
Description
The CSS class to be assigned to the viewport element.
confine variable
Description
Determines if the viewfinder should be confined within the boundary of the target. The viewfinder will not be confined if it is larger than the target itself.
enableScroll variable
Description
When enabled the plugin will track scroll events on the window and animate the viewfinder accordingly. This option will be automatically disabled if mobile is true
or the target lies within a scrollable region which is not the window.
mobile constant
Description
Enable mobile support. Currently all functionality is supported except for enableScroll.
onCreate variable
Description
A callback to be invoked once the plugin has completed it's initialization. See the create event for more details.
onDestroy variable
Description
A callback to be invoked after the plugin has been destroyed. See the destroy event for more details.
onMoveStart variable
Description
A callback to be invoked when the user takes control of the viewfinder. See the moveStart event for more details.
onMoveStop variable
Description
A callback to be invoked when the user cedes control of the viewfinder. See the moveStop event for more details.
onRefresh variable
Description
A callback to be invoked every time the plugin's layout is recalculated. See the refresh event for more details.
smoothing variable
Description
A value on the range 0 to 1, inclusive, which determines how smooth the viewfinder animation is. A value of 0 disables animation while 1 causes the viewfinder to remain stationary.
src constant
Description
The URL of an image resource to be displayed within the plugins viewport.
viewfinder.height variable
Description
Determines the height of the viewfinder when it is presented as a magnifying lens, e.g., no viewport has been specified. A percentage based height is applied relative to the height of the plugin target
viewfinder.hide.duration variable
Description
The duration of the viewfinder's hide animation in milliseconds.
viewfinder.hide.event constant
Description
The trigger event for the viewfinder's hide animation.
viewfinder.move.event constant
Description
The event used to determine the position of the viewfinder. The event type must produce an event
object with pageX
and pageY
properties.
viewfinder.moveStart.event constant
Description
The event which grants control of the viewfinder to the user. Once triggered the event will not be triggered until after the event set by viewfinder.moveStop.event has occurred.
viewfinder.moveStart.jumpTo variable
Description
If true
the viewfinder will jump to the users position when the viewfinder.moveStart.event event is triggered, else the viewfinder is animated to the users position from it's previous position
viewfinder.moveStop.event constant
Description
The event upon which the user cedes control of the viewfinder.
viewfinder.show.duration variable
Description
The duration of the viewfinder's show animation in milliseconds.
viewfinder.show.event constant
Description
The trigger event for the viewfinder to be shown.
viewport constant
Description
Designates an element as the plugins active viewport. If a collection is specified then the first element is selected.
zoom variable
Description
A decimal representing the percent magnification level.
Plugin Methods
The plugin exposes a collection of API methods for controlling it's behavior. There are two general ways to access these methods:
Invoking plugin methods
Once initialized upon a target the simpleZoom()
method may be used to execute API methods.
To execute an API method the plugin is invoked with the name of the preferred method as the first argument
followed by arguments to be passed along to the API method itself.
For example, the option method may be used to set the zoom option as follows.
$("#selector").simpleZoom() //initialize
.simpleZoom(
"option", //plugin method name
"zoom", //first method argument
0.25 //second method argument
);
[Important] Many plugin methods will generate an error when invoked prior to the create event being triggered.
Invoking instance methods
A reference to the plugin's underlying SimpleZoom
instance is returned by the
getSimpleZoom method allowing for direct invocation of plugin methods.
$("#selector").simpleZoom("getSimpleZoom")
.option("zoom", 0.25) //setter methods can be chained
.setPosition(0, 0);
destroy ( removeEvents )
Description
Destroys the plugin instance.
Syntax
-
removeEvents
If
true
then all plugin events are removed from the target..
getOverlay ( )
Description
Get the element which is overlaid upon the target in order to capture user events.
Syntax
getPosition ( )
Description
Get an object detailing the current position and positional constraints of the viewfinder.
Syntax
- Object
Returns
Property | Type | Description |
---|---|---|
top | number | Distance from the center of the viewfinder to the top of the targets content region. |
left | number | Distance from the center of the viewfinder to the left side of the targets content region. |
minLeft | number | The minimum value of the left property. |
maxLeft | number | The maximum value of the left property. |
minTop | number | The minimum value of the top property. |
maxTop | number | The maximum value of the top property. |
getSimpleZoom ( )
Description
Get an object exposing all API methods of the plugin.
Syntax
Examples
var sz = $(".selector").simpleZoom("getSimpleZoom");
isEnabled ( )
Description
Determine whether or not the plugin is enabled for user interaction.
Syntax
off ( event, handler )
Description
Unbinds a handler for the specified event
. See on for further details.
Syntax
-
event
-
handler
on ( event, handler )
Description
Bind a handler to the specified event
.
Syntax
-
event
A whitespace separated list of events. Events may be specified by either their plugin name, e.g., "create" or by their global name, e.g., "simplezoomcreate"
-
handler
An event handler.
option ( optionName )
Description
Get the value of the specified option.
Syntax
-
optionName
The name of an option.
Examples
Get the viewfinder show animation duration.
var duration = $("#selector").simpleZoom("option", "show").duration;
option ( optionName, value )
Description
Set the value of the specified option. Note that while the value of most options can be updated after the plugin has been initialized there are a few exceptions. For more details, refer to the options documentation.
Syntax
-
optionName
The name of an option.
-
value
The value of the specified option.
Examples
Set a primitive value
Set the scale ratio to be 2:1.
$("#selector").simpleZoom("option", "zoom", 0.25); //set 25% magnification
Merge an object
Set the viewfinder show animation duration to 200. Object are merged into the existing options.
$("#selector").simpleZoom("option", "show", {duration: 200});
refresh ( )
Description
Updates the layout. This method should be invoked whenever the target changes position or is resized. By default a throttled version of this method is invoked whenever the browser window is resized.
Syntax
setPosition ( left, top, noAnimation )
Description
Set the position of the viewfinder's center point relative to the content box of the plugin target. Note that if confine is set to true
then the position will be clamped to ensure that it remains within the content box of the target.
Syntax
-
left
The distance in CSS pixels from the left side of the target's content box to the viewfinder's center.
-
top
The distance in CSS pixels from the top of the target's content box to the viewfinder's center.
-
noAnimation
Determines whether or not the invocation should trigger and animation or jump to the final specified coordinates.
Plugin Events
afterHide
Description
Triggered once the viewfinder has been hidden. The viewfinder may be hidden in response to the user triggering viewfinder.hide.event or by a call to the method.
Examples
Bind a handler using the on plugin method.
$("#selector").simpleZoom("on", "afterHide", function(){
//function body
}
Bind a handler using jQuery.on
$("#selector").on("simplezoomafterhide", function(){
//function body
}
afterShow
Description
Triggered after the viewfinder has been shown. The viewfinder may be shown in response to the user triggering viewfinder.show.event or by a call to the method.
beforeDestroy
Description
Triggered before the plugin's generated elements are removed and it's associated SimpleZoom instance is destroyed.
beforeHide
Description
Triggered before the viewfinder is hidden. Typically suceeded by afterHide.
beforeShow
Description
Triggered before the viewfinder is shown. Typically suceeded by afterShow.
create
Description
Triggered once the plugin has been fully initialized. Prior to this event most plugin method calls will be rejected.
destroy
Description
Triggered when the plugin is destroyed. This event may occur in response to the destroy method or prior to the plugin being re-initialized on a previously initialized target.
moveStart
Description
Triggered in response to the user taking control of the viewfinder. Typically this event will occur in response to the event described by the viewfinder.moveStop.event option. Once triggeredthe event will not occur again until after moveStop.
moveStop
Description
Triggered in response to the user losing control of the viewfinder.
refresh
Description
Triggered whenever the plugin layout is updated.
Examples
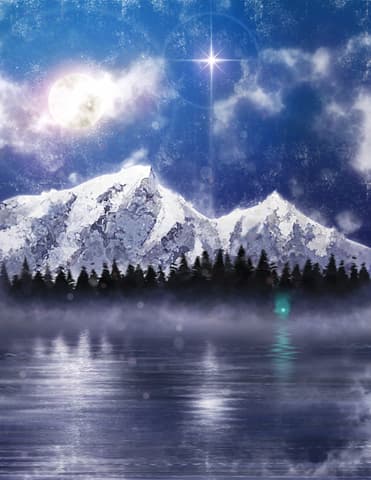
- Magnifying Lens