SimpleZoom: A jQuery Plugin for Magnifying Images
Hello World!
This post refers to an old version of the SimpleZoom plugin. For the latest version of this plugin check out /blog/tag/simplezoom.
For my inaugural blog post I have written a simple image magnifying jQuery plugin known as SimpleZoom. Invoking SimpleZoom upon a target image element will bind a set of functions to the target's mouseover handler. Triggering a mouseover event upon the target will then cause an animated 'lens' element to appear, displaying some portion of a higher resolution version of the target image. Of course, the image data of the higher resolution version must be specified as arguments of SimpleZoom. Complete invocation methods are detailed below.
Compatibility
It is important to note that this first version of SimpleZoom (0.1.0) has a couple limitations in terms of compatibility. While the plugin has been tested to accurately display the magnified image as far back as IE7, the magnifying lens widget will appear as a simple box on non-CSS3 browsers. Additionally, though I don't believe this to be much of a compatibility issue, SimpleZoom does makes use of requestAnimationFrame via a cross-browser function compiled by Paul Irish.
SimpleZoom was built for jQuery 1.7.2
Usage
Given a static image with id='small-img' and a higher resolution version of said image with id='large-img', both of which are already loaded, the plugin method $.fn.simpleZoom can be invoked as follows:
- JavaScript
$('#small-img').simpleZoom('#large-img');
As an alternative to using actual image elements, the SimpleZoom method may be invoked on any static element (e.g. id='small-ele') with a set background image, passing as arguments the URL and dimensions of a higher resolution version of that background image. Note that this method should work even if the call is made before the associated image data has been served up.
To invoke SimpleZoom in this manner set the first argument to null and pass as the second argument an object with a src parameter, specifying the location of an image, along with the parameters maxHeight and maxWidth , specifying the dimensions of the image:
- JavaScript
$('#small-ele').simpleZoom(null,{'maxWidth':900,'maxHeight':500,'src':'larger-img.jpg'});
For fixed dimensional images within a fluid layout SimpleZoom should properly adjust to changes in the images position within the document. However, if the plug-in breaks with the occurrence of some known event, it is possible to obtain a set of helper functions to rectify the issue. By passing 'returnUtil' : true into SimpleZoom as a parameter of the optional secondary argument, the SimpleZoom method will return an object with references to two helper functions called refresh and destruct.
- JavaScript
var util = $('#small-img').simpleZoom($('#large-img'),{'returnUtil':true});
util.refresh();
util.destruct();
A call to util.refresh() alerts SimpleZoom to possible changes in the size and position of the target element, while util.destruct() removes the plug-in completely.
Loading Images
It is sometimes difficult to ensure the proper execution of a function which relies on image data, however, I have found the following method using jQuery to be quite reliable (barring any strange caching issues).
Consider within the document a regular image, labeled as id='small', on which SimpleZoom is to be invoked:
- HTML
<img id='small' src='small.jpg' />
Given the URL of a higher resolution version of small.jpg, SimpleZoom may be safely invoked on $('#small') by employing the following script. Note that in order to ensure proper execution this script should be included either directly after the <img> tags or within a $(document).ready() block:
- JavaScript
var small = $('#small');
var large = $(new Image());
var count = 0;
var callback = function(){
if(++count >= 2){
small.simpleZoom(large);
}
};
large.load(callback).attr('src','large.jpg');
if(small.prop('complete')){
callback();
} else {
small.load(callback);
}
Options
A custom options object may be passed to SimpleZoom as an optional secondary argument. The parameters of this options object can be used to specify the appearance and functionality of the plug-in. For example, as of version 0.1.0 there are three parameters used to specify the size and shape of the 'lens' element, namely, zoomRadius, zoomWidth and zoomHeight . Thus, in order to instantiate SimpleZoom with a circular 'lens' of radius 100 the options object could be constructed as follows.
- JavaScript
$('#small-img').simpleZoom('#large-img',
{ 'zoomRadius' : 100,
'zoomWidth' : 200,
'zoomHeight ': 200 }
);
The full set of valid parameters is listed within the defaults object in the source.
Demo
Mouseover the image below.
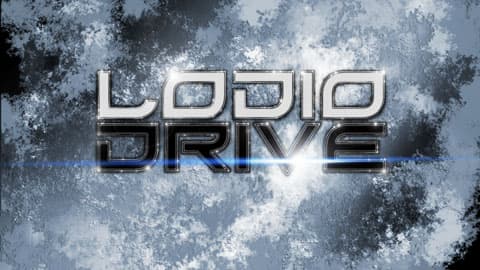